Why you should automatically generate interests from user funds
How to integrate Aave and similar systems in your contracts
If you're writing contracts that use, hold or manage user funds, you might want to consider using those funds for generating free extra income. What's the catch?
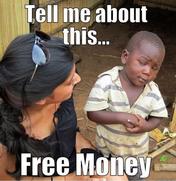
That's right, it's basically free money and leaving funds unused in a contract is wasting a lot of potential. The way these lender/borrower systems work is quite simple conceptually. Once you understand the mechanism and risk involved, you can decide if it's worth it for you or not.
How those Defi markets work
The lending platforms have significantly risen over the last 1-2 years. The core idea in all of them is the same even though the implementations might differ a lot. We will be using Aave mostly as example, but you may have heard of other platforms as well such as Maker, Compound, dYdX, BlockFi or Curve.
Aave is an Ethereum-based peer to peer money market protocol for different tokens. This means you can either borrow a token on Aave or lend it. All borrowers are paying a fee to the lenders and that's where the interests come from. The exact rates can vary and users can choose between stable or variable rates.
In return for lending tokens the lenders receive a respective interest-earning version of the token, e.g., aTokens in Aave or cTokens in Compound. In Aave your aToken balance will automatically increase over time and you can later trade back 1 aToken to 1 original token. That's in contrast to Compound where simply the exchange rate back to the original token changes over time, but your cToken balance won't change. The result will be the same, but the smart contract integration will be slightly different.
What about the latest trend of yield farming?
You might heard of yield farming and the Compound's COMP token. If you are lending out money or borrowing money you will receive the COMP token for free on top. Similarly Aave has a new token coming up. They are intended to be used for governance for the protocol, but you could also just sell them. How to integrate those depends on the system. Since Aave doesn't have a working token for it yet, this goes beyond the scope of the article, but if you want to optimize every little bit of income, then you want to make sure you retrieve those as well once it's possible.
What happens if a borrower runs out of money?
You can imagine that at some point the collateral of a borrower might loose too much in value. Once it drops below whatever the borrowed amount is worth, this can become a problem.
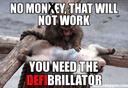
That's why those positions will be automatically liquidated. If a liquidation happens too late it could be a potential security problem. To prevent such a scenario, there exist automated liquidator bots. Great, now we know how it works, but how can you actually use it?
How to integrate Aave
- https://app.aave.com/ for the mainnet
- https://testnet.aave.com/ for the testnet version.
The web app allows you to manually deposit or borrow tokens and is a great way to get your first experience.
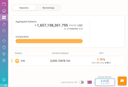
Integrating it into smart contracts
- Call the
deposit
function in Aave and send along any user funds - At some point later call the
redeem
function to convert funds back.
The example uses the Kovan testnet addresses, for more aToken addresses or other networks look at https://docs.aave.com/developers/deployed-contracts/deployed-contract-instances.
pragma solidity >=0.4.22 <0.7.0;
import 'https://github.com/OpenZeppelin/openzeppelin-contracts/blob/master/contracts/token/ERC20/IERC20.sol';
interface IaToken {
function balanceOf(address _user) external view returns (uint256);
function redeem(uint256 _amount) external;
}
interface IAaveLendingPool {
function deposit(address _reserve, uint256 _amount, uint16 _referralCode) external;
}
contract AaveExample {
IERC20 public dai = IERC20(0xFf795577d9AC8bD7D90Ee22b6C1703490b6512FD);
IaToken public aToken = IaToken(0x58AD4cB396411B691A9AAb6F74545b2C5217FE6a);
IAaveLendingPool public aaveLendingPool = IAaveLendingPool(0x580D4Fdc4BF8f9b5ae2fb9225D584fED4AD5375c);
mapping(address => uint256) public userDepositedDai;
constructor() public {
dai.approve(address(aaveLendingPool), type(uint256).max);
}
function userDepositDai(uint256 _amountInDai) external {
userDepositedDai[msg.sender] = _amountInDai;
require(dai.transferFrom(msg.sender, address(this), _amountInDai), "DAI Transfer failed!");
aaveLendingPool.deposit(address(dai), _amountInDai, 0);
}
function userWithdrawDai(uint256 _amountInDai) external {
require(userDepositedDai[msg.sender] >= _amountInDai, "You cannot withdraw more than deposited!");
aToken.redeem(_amountInDai);
require(dai.transferFrom(address(this), msg.sender, _amountInDai), "DAI Transfer failed!");
userDepositedDai[msg.sender] = userDepositedDai[msg.sender] - _amountInDai;
}
}
- Deposit all user DAI directly to Aave.
- If a user want to withdraw funds, we call
redeem
on the aToken.
As you can see it's fairly simple. There is one important aspect missing though:
What to do with the interests
Remember that Aave automatically increases the aToken balance. So if we allow a user to only withdraw what they deposited, we just loose all the extra aTokens since they are now stuck in our contract. You have two options how you could handle this:
- Use all extra interests and do something with them.
- Make a raffle (looking at PoolTogether) or some other fun way to distribute the funds.
- Donate them to charity.
- Pay it out to yourself. You could make your app completely for free and just use the money from the interests as payment.
Or return them fairly to users. One fun way you could achieve this is by minting new tokens that represent ownership of deposited funds in your contracts. For any withdrawal a user burns the tokens again. Then you can see how much of the total supply those tokens are and refund them an equal share of the current aToken balance.
Know that redeems can fail
If you read the Aave documentation for the redeem
function, it states
redeem() will fail if the aTokens to be redeemed are being used as collateral. Please refer to the transferAllowed() function to understand how to check if a specific redeem/transfer action can be performed.
How likely this is to fail depends on the specific market. For any big markets like DAI on Aave this should not happen very often. But if time is critical in your system, you should think about this case. The redeem will work at a later point in time again once the market has sufficient liquidity.
How to choose a market
Well this really depends and everything comes with a trade-off. For a good overview of lending rates, check out the 30 Day Averages on https://defirate.com/lend/.
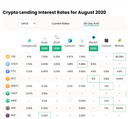
A future of possibilities
Those new markets enable completely new business models that weren't thinkable before. We are living in exciting times and new ideas come up literally every week. It's no better time to start your own idea than now!
I actually have been involved in one new project myself. It hasn't been officially announced yet, so I can't give away too much. But you may want to look at https://magicbet.io/ (placeholder website should hopefully be working by the time you read this) and keep an eye open for it. Maybe you can already guess what it's all about after reading the post and given its name. ;)
Solidity Developer