Advancing the NFT standard: ERC721-Permit
And how to avoid the two step approve + transferFrom with ERC721-Permit (EIP-4494)
There's a new standard in the making. To understand how this really works, I recommend you take a look at my tutorials on:
But we'll try to cover the basics here also. You might be familiar already with ERC20-Permit (EIP-2612). It adds a new permit
function. A user can sign an ERC20 approve
transaction off-chain producing a signature that anyone could use and submit to the permit function. When permit is executed, it will execute the approve
function. This allows for meta-transaction support of ERC20 transfers, but it also simply gets rid of the annoyance of needing two transactions: approve and transferFrom. Now you submit the signature to the smart contract which will call permit
and then transferFrom
in the same transaction.
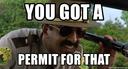
ERC721-Permit: Preventing Misuse and Replays
The main issue we are facing is that a valid signature might be used several times or in other places where it's not intended to be used in. To prevent this we are adding several parameters. Under the hood we are using the already existing, widely used EIP-712 standard.
Okay I know this is getting confusing, EIP-712 and EIP-721 in one standard, but stay with me. We'll always call EIP-721 only ERC-721 from now on to make it easier.
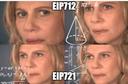
1. EIP-712 Domain Hash
With EIP-712, we define a domain separator for our ERC-721.
bytes32 eip712DomainHash = keccak256(
abi.encode(
keccak256(
"EIP712Domain(string name,string version,uint256 chainId,address verifyingContract)"
),
keccak256(bytes(name())), // ERC-721 Name
keccak256(bytes("1")), // Version
block.chainid,
address(this)
)
);
This ensures a signature is only used for our given token contract address on the correct chain id. The chain id was introduced to exactly identify a network after the Ethereum Classic fork which continued to use a network id of 1. A list of existing chain ids can be seen here.
2. Permit Hash Struct
Now we can create a Permit specific signature:
bytes32 hashStruct = keccak256(
abi.encode(
keccak256("Permit(address spender,uint256 tokenId,uint256 nonce,uint256 deadline)"),
spender,
tokenId,
nonces[tokenId],
deadline
)
);
This hashStruct will ensure that the signature can only used for
- the
permit
function - to approve for
spender
- to approve the given
tokenId
- only valid before the given
deadline
- only valid for the given
nonce
The nonce ensures someone can not replay a signature, i.e., use it multiple times on the same contract. As you can see these are the first real differences to ERC20-Permit. This now works on a tokenId
basis rather than the ERC721 owner address. And also the nonce is only incremented upon transferring an ERC721, not upon calling permit. Why is that?
Well because with permits and NFT's you actually have a unique feature opportunity that's not possible with ERC20. You can allow a user to create multiple permit signatures for multiple spender addresses all for the same tokenId
. All spenders can execute the permit function using the same nonce since we aren't incrementing the nonce inside permit. And only if the NFT is actually transferred, the nonce is incremented making the old signatures invalid. So make sure in your ERC721 contract to increase the nonce for every transfer:
function _transfer(address from, address to, uint256 tokenId) internal override {
_nonces[tokenId]++;
super._transfer(from, to, tokenId);
}
3. Final Hash
bytes32 hash = keccak256(
abi.encodePacked("\x19\x01", eip712DomainHash, hashStruct)
);
4. Verifying the Signature
Using this hash we can use ecrecover to retrieve the signer of the function. But now we come to the next big difference to ERC20-Permit. The signature here is a hash and not the usually used v,r,s signature values. We'll come to why in a second, but first in the normal case you'll need to use assembly to recover the v,r,s values and then use them for the ecrecover:
bytes32 r;
bytes32 s;
uint8 v;
assembly {
r := mload(add(signature, 0x20))
s := mload(add(signature, 0x40))
v := byte(0, mload(add(signature, 0x60)))
}
address signer = ecrecover(hash, v, r, s);
require(signer == owner, "ERC20Permit: invalid signature");
require(signer != address(0), "ECDSA: invalid signature");
Invalid signatures will produce an empty address, that's what the last check is for. So then why do we pass the signature as a string and not as v,r,s values? Seems a little more complicated passing it as string and needing assembly to verify the signature, doesn't it.
Well passing the signature as a string essentially allows for the most flexibility. If the signature is just a string, it's quite easy to extend the verification of it to more than just a regular ecrecover check. In fact already in the ERC721-Permit there are two additional signature verifications included:
EIP-2098 are a compact form standard for signatures. They make use of a mathematical trick in the representation of the signature on the elliptic curve. The details here don't matter, but essentially instead of requiring 65 bytes for a signature, you can represent it with only 64 bytes. But it requires a slightly different signature verification. But if you're using 64 or 65 bytes, obviously in both cases you can represent the signature as a string. Great.
And then EIP-1271 we looked at for meta-transactions already here, but it's essentially a way for smart contracts to create signatures. In our case imagine a smart contract is the owner of the NFT. Usually a smart contract cannot create signatures since there's not private key. When verifying a signature and it it's invalid, in a second step we can check if it's a valid smart contract signature:
We call the owner address of the NFT using staticcall
, which ensures no further state modifications happen in the call. If the result succeeds and has a valid returnData length (this is very critical, see the previous 0x bug), we can check if the return value matches 0x1626ba7e
, the magic value from EIP-1271 which implies 'it's a valid signature'. How the smart contract verifies the signature is up to the contract to decide.
Lastly if you read my post about ecrecover here, you will know there are some issues with it.
Complete Signature Verification
So you should handle those properly. Fortunately doing all of this is easier then it sounds when using the Openzeppelin contracts. A complete signature verification for ERC721-Permit might look like on the right.
First we make use of ECDSA.tryRecover. This will solve a couple things in one:
- Support for EIP-2098 signatures.
- Solving potential security problems with ecrecover.
Then if the signature verification recovers an address, we check if it's the owner of the NFT or if he is approved. You could of course only allow the owner, but since approved addresses in ERC721 should have full control, it's better to also give them control for permits.
And lastly if it's not a valid EOA so far, we can check if it's a valid contract signature. And since a contract can either be directly the owner or approved, we need to check it for both cases using the staticcall functionality.
Note that if a contract is approved via ERC721.setApprovalForAll, we won't be able to verify its signature and allow it to use permit. However, an approved for all contract could simply approve himself first using ERC721.approve.
(address signer, ) = ECDSA.tryRecover(hash, signature);
bool isValidEOASignature = signer != address(0) &&
_isApprovedOrOwner(signer, tokenId);
require(
isValidEOASignature ||
_isValidContractERC1271Signature(
ownerOf(tokenId),
hash, signature
) || _isValidContractERC1271Signature(
getApproved(tokenId),
hash,
signature
),
"ERC721Permit: invalid signature"
);
function _isValidContractERC1271Signature(
address signer,
bytes32 hash,
bytes memory signature
) private view returns (bool) {
(bool success, bytes memory result) = signer.staticcall(
abi.encodeWithSelector(
IERC1271.isValidSignature.selector,
hash,
signature
)
);
return (success &&
result.length == 32 &&
abi.decode(result,(bytes4))
== IERC1271.isValidSignature.selector
);
}
5. Approving the NFT
Now lastly we only have to increase the nonce for the owner and call the approve function:
_approve(owner, spender, amount);
You can see a full implementation example here.
Uniswap ERC721-Permit
The Uniswap implementation has some differences, see implementation here. In Uniswap V3 positions are wrapped in the ERC721 non-fungible token interface and come with permit functinoality.
However notable differences are:
- signarure is passed via v,r,s values
- nonce is incremented upon every permit execution, not allowing multiple permits to be executed
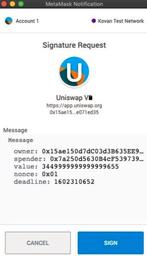
ERC721-Permit Library
I have created an ERC-20 Permit library that you can import. You can find it at https://github.com/soliditylabs/ERC721-Permit.
Built using reference implementation as reference, the way it was meant to be used.
You can simply use it by installing via npm:
$ npm install @soliditylabs/erc721-permit --save-dev
Import it into your ERC-721 contract like this:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import {ERC721, ERC721Permit} from "@soliditylabs/erc20-permit/contracts/ERC20Permit.sol";
contract MyNFTContract is ERC721Permit("MyNFT", "MNFT") {
uint256 private _lastTokenId;
function mint() public {
_mint(msg.sender, ++_lastTokenId);
}
function safeTransferFromWithPermit(
address from,
address to,
uint256 tokenId,
bytes memory _data,
uint256 deadline,
bytes memory signature
) external {
_permit(msg.sender, tokenId, deadline, signature);
safeTransferFrom(from, to, tokenId, _data);
}
}
We also added a safeTransferFromWithPermit
function here. This is not directly a standard function of the ERC721-Permit, but it can still be a pretty useful addition. With that you can approve and transfer in a single call, saving some extra gas and also not requiring any helper contracts.
Frontend Usage
Signing data via EIP-712 is directly supported in a lot of wallets these days. For example in MetaMask take a look here how to integrate it.
As always use with care
Be aware that the standard is not yet final. In fact it's at relatively early draft stage. I will keep the library updated in case the standard changes again. My library code was also not audited, use at your own risk. If you notice any issue or code being outdated, please contact me and I can update it.
You have reached the end of the article. I hereby permit you to comment and ask questions.
Solidity Developer