Integrating Balancer in your contracts
What is Balancer and how to use it
What is Balancer?
Balancer is very similar to Uniswap. If you're not familiar with Uniswap or Balancer yet, they are fully decentralized protocols for automated liquidity provision on Ethereum. An easier-to-understand description would be that they are decentralized exchanges (DEX) relying on external liquidity providers that can add tokens to smart contract pools and users can trade those directly.
Since Balancer is running on Ethereum, what we can trade are Ethereum ERC-20 tokens. Balancer - being fully decentralized - has no restrictions to which tokens can be added or even how many pools can be created per token.
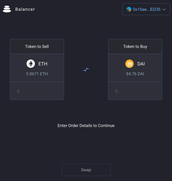
What is different compared to Uniswap?
In Uniswap each pool contains exactly one token pair. That's why it also doesn't make sense to have multiple pools for the same pair. In contrast in Balancer you can have multiple tokens in a single pool with different relations. Take a look at https://pools.balancer.exchange/#/:
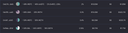
You'll see that a pool can have different balances for each token. What that means for liquidity providers is that they can have exposure to different assets without having to deal with complicated and/or expensive rebalancing procedures over different pools. They can pick or even create a pool that suits their needs and provide liquidity for it.
After each trade the balances per token would usually change and destroy the nicely defined relations in a pool. That's why Balancer (hence the name) makes sure that after each trade a pool will be rebalanced.
How are pools rebalanced?
In each pool there's a multi-dimensional invariant function which defines swap prices between any two tokens in any pool. This is the heart of the mechanism and allows the rebalancing. Given defined prices for each token swap, a pool can be balanced the following:
Imagine a trade happens in a 50:50 DAI/WETH pool. Somebody just purchased DAI in the amount of 1% of the pool's liquidity. Now the pool balances are 49% DAI and 51% WETH. A conventional rebalancing now has to purchase back the missing DAI from some other place usually inferring a fee. Instead in Balancer, rational market actors actively buy WETH from the pool bringing it back to the old ratio. So instead of paying a fee, the pool actually receives fees in this mechanism.
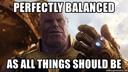
Smart Order Routing (SOR)
The SOR is a conceptual way of dealing with pools to optimize the profit for a trader. They also have an off-chain JavaScript solution available, source code is here. You would use this in the frontend and you can see in the Exchange UI code, that's what they do.
The exact motivation behind SOR is perfectly described in the documentation:
'Since there might be many Balancer pools that contain a given pair, trading only with the most liquid pool is not ideal. The fact that after a one-pool-trade arbitrageurs can profit by levelling all pool prices means the one-pool trader left value on the table.
The ideal solution in a world where there were no gas costs or gas limits would be to trade with all pools available in the desired pair. The amount traded with each pool should move them all to the same new spot price. This would mean all pools would be in an arbitrage free state and no value could be further extracted by arbitrageurs (which always comes at the expense of the trader).
As there are incremental gas costs associated with interacting with each additional Balancer pool, SOR makes sure that the increase in the returns an incremental pool brings exceeds the incremental gas costs. This is an optimization problem that depends therefore on the gas fees paid to the Ethereum network: a higher gas price may cause SOR to select less pools than otherwise.'
Future versions
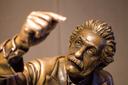
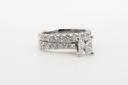
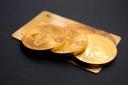
- Bronze: The initial release from earlier this year.
- Silver: Silver is currently in a design phase with an expected release date in late 2020.
- Gold: Final version.
The Silver version will include most notably an on-chain SOR (unless this feature comes only in the Gold release).
Further Balancer resources
Integrating Balancer Bronze into contracts
Now to integrate Balancer, you need to choose a pool or a mix of pools. You could add the SOR feature to find the optimal pools to maximize every bit for the user. This is beyond the scope of the tutorial and not really required if users aren't transferring massive amounts of value.
If you want to do this via SOR, install the @balancer-labs/sor package in the frontend app. Use it to find out the optimal swap strategy. The result will be an array of Swap definitions (how much to trade in which pool). Then you can pass these Swap definitions to the smart contract and execute each one.
We will instead be doing only a single swap. Let's use again DAI and WETH in the Kovan testnet. You can interact with the Kovan contracts in the frontend via:
Let's choose the DAI/WETH/MKR pool: https://kovan.pools.balancer.exchange/#/pool/0x1492b5b01350b7c867185a643f2e59f7be279fd3/. For a mainnet contract, make sure that the pool has very high liquidity if you're using only a single pool. Now let's try and enable ETH payments that are actually converted to DAI, so a user has the choice between paying in DAI or in ETH:
Adding ETH payments as alternative
function pay(uint paymentAmountInDai) public payable {
if (msg.value > 0) {
_swapEthForDai(paymentAmountInDai);
} else {
require(daiToken.transferFrom(msg.sender, address(this), paymentAmountInDai));
}
// do something with that DAI
...
}
A simple check like this at the beginning of your function will be enough. Now as for the _swapEthForDai
function, it will look like something this:
Converting the ETH to DAI
function _swapEthForDai(uint daiAmount) private {
_wrapEth(); // wrap ETH and approve to balancer pool
PoolInterface(bPool).swapExactAmountOut(
address(weth),
type(uint).max, // maxAmountIn, set to max -> use all sent ETH
address(daiToken),
daiAmount,
type(uint).max // maxPrice, set to max -> accept any swap prices
);
require(daiToken.transfer(msg.sender, daiToken.balanceOf(address(this))), "ERR_TRANSFER_FAILED");
_refundLeftoverEth();
}
There are several things to unpack here.
- WETH: You might notice that we are using WETH here. In Balancer there are no direct ETH pairs, all ETH must be converted to WETH first.
swapExactAmountOut
: This function can be used to receive an exact amount of tokens for it. Any leftover tokens not required will be refunded, so make sure you have the fallback function in your contract. If you instead want the other way around to use an exact amount of ETH to pay, use the swapExactAmountIn.- Refund: Once the trade is finished, we can return any leftover ETH to the user. This sends out all ETH from the contract, so if your contract might have an ETH balance for other reasons, make sure to change this.
Wrapping the initial ETH
As for the _wrapEth
function, you simply deposit the the ETH into the WETH contract and approve it for the balancer pool:
function _wrapEth() private {
weth.deposit{ value: msg.value }();
if (weth.allowance(address(this), address(bPool)) < msg.value) {
weth.approve(address(bPool), type(uint).max);
}
}
Refunding leftover ETH
The opposite is happening inside _refundLeftoverEth
, we withdraw any leftover WETH and then return any ETH to the user.
function _refundLeftoverEth() private {
uint wethBalance = weth.balanceOf(address(this));
if (wethBalance > 0) {
// refund leftover ETH
weth.withdraw(wethBalance);
(bool success,) = msg.sender.call{ value: wethBalance }("");
require(success, "ERR_ETH_FAILED");
}
}
And let's not forget to add a fallback function to receive refunded ETH:
receive() external payable {}
And lastly, you'll also need the interface definitions:
interface PoolInterface {
function swapExactAmountIn(address, uint, address, uint, uint) external returns (uint, uint);
function swapExactAmountOut(address, uint, address, uint, uint) external returns (uint, uint);
}
interface TokenInterface {
function balanceOf(address) external returns (uint);
function allowance(address, address) external returns (uint);
function approve(address, uint) external returns (bool);
function transfer(address, uint) external returns (bool);
function transferFrom(address, address, uint) external returns (bool);
function deposit() external payable;
function withdraw(uint) external;
}
What do you think of Balancer? Have you tried it before? Let me know in the comments and ask anything if you need further help.
Solidity Developer