Utilizing Bitmaps to dramatically save on Gas
A simple pattern which can save you a lot of money
As you may know the most expensive operation in Ethereum is storing data (SSTORE). So you should always look for ways to reduce the storage requirements. Let's explore a particularly useful one: Bitmaps.
How to implement a simple Bitmap
Let's assume we want to store 10 boolean values. Usually you would implement this with a simple bool array:
// SPDX-License-Identifier: MIT
pragma solidity 0.8.0;
contract BitmapTest {
bool[10] implementationWithBool;
function setDataWithBoolArray(bool[10] memory data) external {
implementationWithBool = data;
}
function readWithBoolArray(uint256 index) external returns (bool) {
return implementationWithBool[index];
}
}
Now with the Bitmap the implementation can be changed to a uint10
instead of the bool array. The uint10 would be represented by 10 bits in storage.
For example here are some decimals numbers represented in bits.
- 0: 0000000000
- 1: 0000000001
- 512: 0100000000
- 729: 1011011001
- 1023: 1111111111
We can take advantage of this bit-representation with some additional math. To get the n-th bit of this uint, we can use bit-wise operations.
Let's take the number 729. With a bool array to read the 4th bool value, it's just an array[4]
. For the bitmap we can instead create a second number by shifting 1 towards the left using the left-shift operator <<
.
- 1 << 4 = 0000000001 << 4 = 0000010000
Now using the bitwise AND operator &, we get the value of the n-th bit (counting from 0):
- 729 & (1 << 4) = 1011011001 & 0000010000
Which results in
- 1011011001 &
- 0000010000 =
- 0000010000
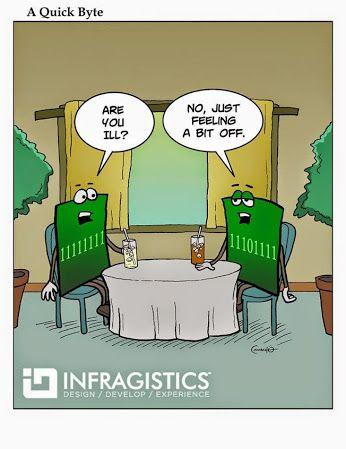
As long as this result is above 0, the n-th bit in the original number was 1. So now we can implement the Bitmap:
// SPDX-License-Identifier: MIT
pragma solidity 0.8.0;
contract BitmapTest {
uint256 implementationWithBitmap;
function setDataWithBitmap(uint256 data) external {
implementationWithBitmap = data;
}
function readWithBitmap(uint256 indexFromRight) external returns (bool) {
uint256 bitAtIndex = implementationWithBitmap & (1 << indexFromRight);
return bitAtIndex > 0;
}
}
Choosing a Bitmap size
As you might have noticed, we chose uint256
in the above implementation. While a uint10
is technically enough, this would actually result in higher gas-costs than using the uint256
. This is because the EVM operates on 32 bytes registers (256 bits) and anything below that requires additional conversion.
So should you always choose uint256
?
No, it depends on your use case. With a single uint256
, you can represent 256 bits. So would the data you want to store fit into a 256 boolean array? If yes then go ahead and use a single uint256
.
If not, for example if the boolean array can grow arbitrarily large, then pack the bitmap itself into an array. We'll explore both options with an example at the end.
Gas Costs Comparisons
Let's first look at the gas costs difference in our 10-bit example. With the original boolean array, the transaction execution costs were:
- setDataWithBoolArray: 140,583 gas
readWithBoolArray
: 1,281 gas
Now with the bitmap, we can greatly improve on that:
setDataWithBitmap
: 78,043 gasreadWithBitmap: 1,129 gas
Use Case 1: Setting Boolean Flags
Now to our first example use case. Boolean flags are commonly used to activate certain options in the system.
Let's say you build a DEX like Uniswap where you have automatically triggered trades. You could activate certain settings depending on where the trade comes from. So for example you could have flags like
NO_FEES
SENDING_FEES_TO_GOVERNANCE
DELAY_TRADE_EXECUTION
The options will likely not exceed 256, so you can easily store those in a single uint256
.
Use Case 2: List of participants
You may want to payout rewards to anyone who has participated in your contracts. This could be an arbitrary large list. You could save each individual participant in a mapping, or instead utilize the bitmap with a uint256 array.
// SPDX-License-Identifier: MIT
pragma solidity 0.8.0;
contract ParticipatedWithBitmap {
uint256[] public participantsBitmap;
function setParticipants(uint256[] memory participantsBitmap_) external onlyOwner {
participantsBitmap = participantsBitmap_;
}
function hasParticipated(uint256 bitmapIndex, uint256 indexFromRight) external view returns (bool) {
uint256 bitAtIndex = participantsBitmap[bitmapIndex] & (1 << indexFromRight);
return bitAtIndex > 0;
}
}
Solidity Developer
More great blog posts from Markus Waas
How to use ChatGPT with Solidity
Using the Solidity Scholar and other GPT tips
Welcome your new best friend as a Solidity developer: ChatGPT. If you're a developer and not using ChatGPT yet, then what the hell are you doing? Let's explore some unique ways this can help you as a Solidity developer. You can just chat directly with ChatGPT or you can use custom GPTs. I have...
How to integrate Uniswap 4 and create custom hooks
Let's dive into Uniswap v4's new features and integration
Uniswap v4 adds several key updates to improve gas efficiency, customizability, and functionality. So let's not lose time and dive into it! By now you've probably heard of Uniswap and so-called AMMs (automated market makers). But if you're not familiar with Uniswap yet, it's a fully decentralized...
How to integrate Wormhole in your smart contracts
Entering a New Era of Blockchain Interoperability
Wormhole began as a token bridge between Ethereum and Solana and has since expanded into a decentralized interoperability protocol for multiple blockchain ecosystems. Wormhole now supports many chains like Ethereum, Cosmos, Polkadot, Injective and many more. It makes cross-chain communication...
Solidity Deep Dive: New Opcode 'Prevrandao'
All you need to know about the latest opcode addition
Let’s back up for a second and figure out what has changed since ‘ The Merge ’. The upgrade finally brought a new consensus mechanism to Ethereum. Instead of the old Proof of Work, blocks are now produced via Proof of Stake . Proof of Work finds consensus via block hashes and a process called...
How Ethereum scales with Arbitrum Nitro and how to use it
A blockchain on a blockchain deep dive
Have you heard of Arbitrum Nitro? The new WAVM enables Plasma but for smart contracts in a super efficient way! It enables having a side chain with guarantees of the Ethereum mainnet chain. Arbitrum has already been one of the most successful Layer 2s so far, and the new Nitro is a major upgrade...
The Ultimate Merkle Tree Guide in Solidity
Everything you need to know about Merkle trees and their future
Most of you probably have heard of Merkle trees by now. They are used everywhere in the world of blockchain. But are you really sure exactly How they work? What the best ways to use them are? What the future holds for Merkle trees? This is not a Merkle tree. What are Merkle Trees? Ralph Merkle...
The New Decentralized The Graph Network
What are the new features and how to use it
Quite some time has passed since my last post about The Graph. If you don't know what it is and why it's useful, go and read the post. It's still relevant and explains in detail why it's needed and how to use it with the centralized hosted service. But the tl;dr is: Events on a blockchain are a...
zkSync Guide - The future of Ethereum scaling
How the zero-knowledge tech works and how to use it
Have you heard of zkSync and its new zkEVM? The new zkSync EVM enables Zero-knowledge proofs for any smart contract executions. What does that mean? Well read on later. But what it enables is having a side chain with similar (not not exact) guarantees of the Ethereum mainnet chain. How cool is...
Exploring the Openzeppelin CrossChain Functionality
What is the new CrossChain support and how can you use it.
For the first time Openzeppelin Contracts have added CrossChain Support. In particular the following chains are currently supported: Polygon: One of the most popular sidechains right now. We've discussed it previously here. Optimism: A Layer 2 chain based on optimistic rollups. We discussed the...
Deploying Solidity Contracts in Hedera
What is Hedera and how can you use it.
Hedera is a relatively new chain that exists since a few years, but recently added token service and smart contract capabilities. You can now write and deploy Solidity contracts to it, but it works a little differently than what you might be used to. Let's take a look! What is the Hedera Network?...
Writing ERC-20 Tests in Solidity with Foundry
Blazing fast tests, no more BigNumber.js, only Solidity
Maybe you are new to programming and are just starting to learn Solidity? One annoyance for you might have been that you were basically required to learn a second language (JavaScript/TypeScript) to write tests. This was undoubtedly a downside which is now gone with the new foundry framework. But...
ERC-4626: Extending ERC-20 for Interest Management
How the newly finalized standard works and can help you with Defi
Many Defi projects have an ERC-20 token which represents ownership over an interest generating asset. This is for example the case for lending/borrowing platforms (money markets) like Compound and Aave. As a lender you will receive aDAI or cDAI. And since lenders receive interest payments for...
Advancing the NFT standard: ERC721-Permit
And how to avoid the two step approve + transferFrom with ERC721-Permit (EIP-4494)
There's a new standard in the making. To understand how this really works, I recommend you take a look at my tutorials on: ERC721 ERC20-Permit ecrecover incl EIP712 But we'll try to cover the basics here also. You might be familiar already with ERC20-Permit (EIP-2612). It adds a new permit...
Moonbeam: The EVM of Polkadot
Deploying and onboarding users to Moonbeam or Moonriver
We've covered several Layer 2 sidechains before: Polygon xDAI Binance Smart Chain Evmos Aurora (NEAR) But Moonbeam is unique since it's a parachain of the Polkadot ecosystem. It only just launched which means you are now able to deploy smart contracts to the chain. Being able to deploy EVM...
Advanced MultiSwap: How to better arbitrage with Solidity
Making multiple swaps across different decentralized exchanges in a single transaction
If you want maximum arbitrage performance, you need to swap tokens between exchanges in a single transaction. Or maybe you just want to save gas on certain swaps you perform regularly. Or maybe you have your own custom use case for swapping between decentralized exchanges. And of course maybe you...
Deploying Solidity Smart Contracts to Solana
What is Solana and how can you deploy Solidity smart contracts to it?
Solana is a new blockchain focusing on performance. It supports smart contracts like Ethereum which they call Programs. You can develop those in Rust, but there's also a new project now to compile Solidity to Solana. In other words you can deploy your contracts written in Solidity now to Solana!...
Smock 2: The powerful mocking tool for Hardhat
Features of smock v2 and how to use them with examples
We’ve covered mocking contracts before as well as the first version of the new mocking tool Smock 2. It simplifies the mocking process greatly and also gives you more testing power. You’ll be able to change the return values for functions as well as changing internal contract storage directly!...
How to deploy on Evmos: The first EVM chain on Cosmos
Deploying and onboarding users to Evmos
We've covered several Layer 2 sidechains before: Polygon xDAI Binance Smart Chain Aurora Chain (NEAR) Optimism But this time we will do into the exciting new world of Cosmos. Many of the most interesting projects are currently building in the ecosystem and you can expect a lot to happen here in...
EIP-2535: A standard for organizing and upgrading a modular smart contract system.
Multi-Facet Proxies for full control over your upgrades
The EIP-2535 standard has several projects already using it, most notably Aavegotchi holding many millions of dollars. What is it and should you use it instead of the commonly used proxy upgrade pattern? What is a diamond? We're not talking about diamond programmer hands here of course. A diamond...
MultiSwap: How to arbitrage with Solidity
Making multiple swaps across different decentralized exchanges in a single transaction
If you want maximum arbitrage performance, you need to swap tokens between exchanges in a single transaction. Or maybe you just want to save gas on certain swaps you perform regularly. Or maybe you have your own custom use case for swapping between decentralized exchanges. And of course maybe you...
The latest tech for scaling your contracts: Optimism
How the blockchain on a blockchain works and how to use it
Have you heard of Optimism? The new Optimistic VM enables Plasma but for smart contracts! What does that mean? Well read on. But what it enables is having a side chain with guarantees of the Ethereum mainnet chain. How cool is that? And you can already use it for several apps on mainnet....
Ultimate Performance: The Aurora Layer2 Network
Deploying and onboarding users to the Aurora Network powered by NEAR Protocol
We've covered several Layer 2 sidechains before: Polygon xDAI Binance Smart Chain But today might be the fastest of them all. On top it's tightly connected to the NEAR protocol ecosystem, a PoS chain with a scalable sharding design. And of course they have a bridge to Ethereum! What is the Aurora...
What is ecrecover in Solidity?
A dive into the waters of signatures for smart contracts
Ever wondered what the hell the deal is with the ecrecover command in Solidity? It's all about signatures and keys... What is ecrecover ? You may have seen ecrecover in a Solidity contract before and wondered what exactly the deal with this was. Well you came across the EVM precompile ecrecover....
How to use Binance Smart Chain in your Dapp
Deploying and onboarding users to the Binance Smart Chain (BSC)
Defi has been a major contributor to the Binance Smart Chain taking off recently. Along with increasing gas costs on Ethereum mainnet which are actually at one of the lowest levels since a long time at the time of this writing, but will likely pump again at the next ETH price pump. So how does...
Using the new Uniswap v3 in your contracts
What's new in Uniswap v3 and how to integrate Uniswap v3
If you're not familiar with Uniswap yet, it's a fully decentralized protocol for automated liquidity provision on Ethereum. An easier-to-understand description would be that it's a decentralized exchange (DEX) relying on external liquidity providers that can add tokens to smart contract pools and...
What's coming in the London Hardfork?
Looking at all the details of the upcoming fork
The Berlin Hardfork only just went live on April 14th after block 12,224,00. Next up will be the London Hardfork in July which will include EIP-1559 and is scheduled for July 14th (no exact block decided yet). So let's take a look at the new changes and what you need to know as a developer....
Welcome to the Matrix of blockchain
How to get alerted *before* getting hacked and prevent it
Defi hacks alone have totaled $285M just since 2019. Let's take the Balancer hack for example. The hack was exploiting the fact that a pool with a deflationary token STA (Statera) was created. The pool accumulated a significant liquidity when it was eventually drained by the hack. Read my post on...
The Ultimate Ethereum Mainnet Deployment Guide
All you need to know to deploy to the Ethereum mainnet
We all love Ethereum, so you've built some great smart contracts. They are tested intensely with unit-tests and on testnets. Now it's finally time to go to mainnet. But this is a tricky business... 1. What exactly is a deployment transaction? First let's quickly discuss what a contract deployment...
SushiSwap Explained!
Looking at the implementation details of SushiSwap
You've probably heard of SushiSwap by now. The Uniswap fork brought new features like staking and governance to the exchange. But how exactly are the contracts behind it working? It's actually not too difficult. Knowing how this works in detail will be a great way to learn about Solidity and...
Solidity Fast Track 2: Continue Learning Solidity Fast
Continuing to learn Solidity fast with the advanced basics
Previously we learned all of the basics in 20 minutes. If you are a complete beginner, start there and then come back here. Now we'll explore some more advanced concepts, but again as fast as possible. 1. Saving money with events We all know gas prices are out of control right now, so it's more...
What's coming in the Berlin Hardfork?
Looking at all the details of the upcoming fork
The Berlin Hardfork is scheduled for April 14th after block 12,224,00. Later to be followed by the London Hardfork in July which will include EIP-1559. So let's take a look at the new changes and what you need to know as a developer. EIP-2929: Increased gas costs for state access EIP-2929 will...
Using 1inch ChiGas tokens to reduce transaction costs
What are gas tokens and example usage for Uniswap v2
Gas prices have been occasionally above 1000 Gwei in the past in peak times. Given an ETH price of over 1000 USD, this can lead to insane real transaction costs. In particular this can be a pain when using onchain DEX's like Uniswap, resulting in hundreds of dollars transaction fees for a single...
Openzeppelin Contracts v4 in Review
Taking a look at the new Openzeppelin v4 Release
The Openzeppelin v4 contracts are now available in Beta and most notably come with Solidity 0.8 support. For older compiler versions, you'll need to stick with the older contract versions. The beta tag means there still might be small breaking changes coming for the final v4 version, but you can...
EIP-3156: Creating a standard for Flash Loans
A new standard for flash loans unifying the interface + wrappers for existing ecosystems
As we've discussed last week, flash loans are a commonly used pattern for hacks. But what exactly are they and how are they implemented in the contracts? As of right now each protocol has its own way of implementing flash loans. With EIP-3156 we will get a standardized interface. The standard was...
Tornado.cash: A story of anonymity and zk-SNARKs
What is Tornado.cash, how to use it and the future
With the recent Yearn vault v1 hack from just a few days ago, we can see a new pattern of hacks emerging: Get anonymous ETH via tornado.cash. Use the ETH to pay for the hack transaction(s). Use a flash loan to decrease capital requirements. Create some imbalances given the large capital and...
High Stakes Roulette on Ethereum
Learn by Example: Building a secure High Stakes Roulette
It's always best to learn with examples. So let's build a little online casino on the blockchain. We'll also make it secure enough to allow playing in really high stakes by adding a secure randomness generator. Let's discuss the overall design first. Designing the contract Before we program...
How to implement generalized meta transactions
We'll explore a powerful design for meta transactions based on 0x
Enabling meta transactions inside your contract is a powerful addition. Requiring users to hold ETH to pay for gas has always been and still is one of the biggest user onboarding challenges. Who knows how many more people would be using Ethereum right now if it was just a simple click? But...
Using the new Uniswap v2 as oracle in your contracts
How does the Uniswap v2 oracle function and how to integrate with it
We've covered Uniswap previously here. But let's go through the basics first again. What is UniSwap? If you're not familiar with Uniswap yet, it's a fully decentralized protocol for automated liquidity provision on Ethereum. An easier-to-understand description would be that it's a decentralized...
Smock: The powerful mocking tool for Hardhat
Features of smock and how to use them with examples
We’ve covered mocking contracts before, but now there’s an additional great tool available: smock. It simplifies the mocking process greatly and also gives you more testing power. You’ll be able to change the return values for functions as well as changing internal contract storage directly! How...
How to build and use ERC-721 tokens in 2021
An intro for devs to the uniquely identifying token standard and its future
The ERC-721 standard has been around for a while now. Originally made popular by blockchain games, it's more and more used for other applications like Defi. But what exactly is it? A non-fungible token (NFT) is a uniquely identifying token. The word non-fungible implies you cannot just replace...
Trustless token management with Set Protocol
How to integrate token sets in your contracts
With Set Protocol you can create baskets of tokens that give users different levels of exposure to underlying assets (currently only ERC-20 tokens). Set Protocol and their TokenSet functionality is the perfect example for making use of the new paradigm of Defi and composability. You can let...
Exploring the new Solidity 0.8 Release
And how to upgrade your contracts to Solidity 0.8
We are getting closer to that Solidity 1.0 release (unless of course after 0.9 comes 0.10). Now Solidity 0.8 has been released only 5 months after the 0.7 release! Let's explore how you can migrate your contracts today... New features & how to use them Let's look at the two big new features which...
How to build and use ERC-1155 tokens
An intro to the new standard for having many tokens in one
ERC-1155 allows you to send multiple different token classes in one transaction. You can imagine it as transferring Chinese Yuan and US Dollars in a single transfer. ERC-1155 is most commonly known for being used in games, but there are many more use cases for it. First of all though, what are...
Leveraging the power of Bitcoins with RSK
Learn how RSK works and how to deploy your smart contracts to it
I'm always interested in what other ways one can use their blockchain and Solidity skills. While many projects are still only in the planning or in testnet status, with Rootstock (RSK) you can transfer mainnet Bitcoins to an EVM sidechain and vice-versa already today. Utilizing the power of the...
Solidity Fast Track: Learn Solidity Fast
'Learn X in Y minutes' this time with X = Solidity 0.7 and Y = 20
You might be familiar with the Learn X in Y minutes. For example you could learn JavaScript in 20 minutes at https://learnxinyminutes.com/docs/javascript/. Unfortunately there is no equivalent for Solidity, but this is about to change. Do you have 20 minutes to learn all of the basics? We even...
Sourcify: The future of a Decentralized Etherscan
Learn how to use the new Sourcify infrastructure today
We all love Etherscan. It's a great tool to interact with contracts, read the source codes or just see the status of your transactions. But unfortunately as great as it is, we should not forget that it's a centralized service. The website could be taken down any day. This kind of defeats the...
Integrating the 0x API into your contracts
How to automatically get the best prices via 0x
How can you add 0x to your contracts to automatically convert between tokens? We have done this in a similar fashion before with Uniswap and Balancer. The 0x API has a bit of a twist. Let's take a look why... Why you want 0x in your contracts? It's simple: Okay, but seriously. Let's see why the...
How to build and use ERC-777 tokens
An intro to the new upgraded standard for ERC-20 tokens
The new upgraded standard for ERC-20 tokens is becoming more and more popular. It's fully backwards compatible, you can easily create one using the Openzeppelin contracts and there are many interesting new features not available in ERC-20. Should you upgrade from ERC-20? Well let's look into what...
COMP Governance Explained
How Compound's Decentralized Governance is working under the hood
You might have heard about the COMP token launch. With a current market cap of over 350 million USD, the token has accumulated massive value. But what is the actual utility of COMP? It's a governance token. Compound being a fully decentralized system (or at least on the way towards it), has a...
How to prevent stuck tokens in contracts
And other use cases for the popular EIP-165
Do you remember the beginning of the Dark Forest story? If not, let's look at it again: Somebody sent tokens to a smart contract that was not intended to receive tokens. This perfectly illustrates one of the issues not only with ERC-20 tokens, but generally with smart contracts. How can we find...
Understanding the World of Automated Smart Contract Analyzers
What are the best tools today and how can you use them?
As we all know, it's very difficult writing a complex, yet fully secure smart contract. Without the proper methods, chances are you will have many security issues. Automated security testing tools already exist and can be a great help. One of the main challenges for these tools is to maximize...
A Long Way To Go: On Gasless Tokens and ERC20-Permit
And how to avoid the two step approve + transferFrom with ERC20-Permit (EIP-2612)!
It's April 2019 in Sydney. Here I am looking for the Edcon Hackathon inside the massive Sydney university complex. It feels like a little city within a city. Of course, I am at the wrong end of the complex and I realize to get to the venue hosting the Hackathon I need to walk 30 minutes to the...
Smart Contract Testing with Waffle 3
What are the features of Waffle and how to use them.
Waffle has been a relatively recent new testing framework, but has gained a lot of popularity thanks to its simplicity and speed. Is it worth a try? Absolutely. I wouldn't run and immediately convert every project to it, but you might want to consider it for new ones. It's also actively being...
How to use xDai in your Dapp
Deploying and onboarding users to xDai to avoid the high gas costs
Gas costs are exploding again, ETH2.0 is still too far away and people are now looking at layer 2 solutions. Here's a good overview of existing layer 2 projects: https://github.com/Awesome-Layer-2/awesome-layer-2. Today we will take a closer look at xDai as a solution for your Dapp. What are...
Stack Too Deep
Three words of horror
You just have to add one tiny change in your contracts. You think this will take you only a few seconds. And you are right, adding the code took you less than a minute. All happy about your coding speed you enter the compile command. With such a small change, you are confident your code is...
Integrating the new Chainlink contracts
How to use the new price feeder oracles
By now you've probably heard of Chainlink. Maybe you are even participating the current hackathon? In any case adding their new contracts to retrieve price feed data is surprisingly simple. But how does it work? Oracles and decentralization If you're confused about oracles, you're not alone. The...
TheGraph: Fixing the Web3 data querying
Why we need TheGraph and how to use it
Previously we looked at the big picture of Solidity and the create-eth-app which already mentioned TheGraph before. This time we will take a closer look at TheGraph which essentially became part of the standard stack for developing Dapps in the last year. But let's first see how we would do...
Adding Typescript to Truffle and Buidler
How to use TypeChain to utilize the powers of Typescript in your project
Unlike compiled languages, you pretty much have no safeguards when running JavaScript code. You'll only notice errors during runtime and you won't get autocompletion during coding. With Typescript you can get proper typechecking as long as the used library exports its types. Most Ethereum...
Integrating Balancer in your contracts
What is Balancer and how to use it
What is Balancer? Balancer is very similar to Uniswap. If you're not familiar with Uniswap or Balancer yet, they are fully decentralized protocols for automated liquidity provision on Ethereum. An easier-to-understand description would be that they are decentralized exchanges (DEX) relying on...
Navigating the pitfalls of securely interacting with ERC20 tokens
Figuring out how to securely interact might be harder than you think
You would think calling a few functions on an ERC-20 token is the simplest thing to do, right? Unfortunately I have some bad news, it's not. There are several things to consider and some errors are still pretty common. Let's start with the easy ones. Let's take a very common token: ... Now to...
Why you should automatically generate interests from user funds
How to integrate Aave and similar systems in your contracts
If you're writing contracts that use, hold or manage user funds, you might want to consider using those funds for generating free extra income. What's the catch? That's right, it's basically free money and leaving funds unused in a contract is wasting a lot of potential. The way these...
How to use Polygon (Matic) in your Dapp
Deploying and onboarding users to Polygon to avoid the high gas costs
Gas costs are exploding again, ETH2.0 is still too far away and people are now looking at layer 2 solutions. Here's a good overview of existing layer 2 projects: https://github.com/Awesome-Layer-2/awesome-layer-2. Today we will take a closer look at Polygon (previously known as Matic) as a...
Migrating from Truffle to Buidler
And why you should probably keep both.
Why Buidler? Proper debugging is a pain with Truffle. Events are way too difficult to use as logging and they don't even work for reverted transactions (when you would need them most). Buidler gives you a console.log for your contracts which is a game changer. And you'll also get stack traces...
Contract factories and clones
How to deploy contracts within contracts as easily and gas-efficient as possible
The factory design pattern is a pretty common pattern used in programming. The idea is simple, instead of creating objects directly, you have an object (the factory) that creates objects for you. In the case of Solidity, an object is a smart contract and so a factory will deploy new contracts for...
How to use IPFS in your Dapp?
Using the interplanetary file system in your frontend and contracts
You may have heard about IPFS before, the Interplanetary File System. The concept has existed for quite some time now, but with IPFS you'll get a more reliable data storage, thanks to their internal use of blockchain technology. Filecoin is a new system that is incentivizing storage for IPFS...
Downsizing contracts to fight the contract size limit
What can you do to prevent your contracts from getting too large?
Why is there a limit? On November 22, 2016 the Spurious Dragon hard-fork introduced EIP-170 which added a smart contract size limit of 24.576 kb. For you as a Solidity developer this means when you add more and more functionality to your contract, at some point you will reach the limit and when...
Using EXTCODEHASH to secure your systems
How to safely integrate anyone's smart contract
What is the EXTCODEHASH? The EVM opcode EXTCODEHASH was added on February 28, 2019 via EIP-1052. Not only does it help to reduce external function calls for compiled Solidity contracts, it also adds additional functionality. It gives you the hash of the code from an address. Since only contract...
Using the new Uniswap v2 in your contracts
What's new in Uniswap v2 and how to integrate Uniswap v2
Note : For Uniswap 3 check out the tutorial here. What is UniSwap? If you're not familiar with Uniswap yet, it's a fully decentralized protocol for automated liquidity provision on Ethereum. An easier-to-understand description would be that it's a decentralized exchange (DEX) relying on external...
Solidity and Truffle Continuous Integration Setup
How to setup Travis or Circle CI for Truffle testing along with useful plugins.
Continuous integration (CI) with Truffle is great for developing once you have a basic set of tests implemented. It allows you to run very long tests, ensure all tests pass before merging a pull request and to keep track of various statistics using additional tools. We will use the Truffle...
Upcoming Devcon 2021 and other events
The Ethereum Foundation just announced the next Devcon in 2021 in Colombia
Biggest virtual hackathon almost finished First of all, the current HackMoney event has come to an end and it has been a massive success. One can only imagine what kind of cool projects people have built in a 30 days hackathon. All final projects can be seen at:...
The Year of the 20: Creating an ERC20 in 2020
How to use the latest and best tools to create an ERC-20 token contract
You know what an ERC-20 is, you probably have created your own versions of it several times (if not, have a look at: ERC-20). But how would you start in 2020 using the latest tools? Let's create a new ERC-2020 token contract with some basic functionality which focuses on simplicity and latest...
How to get a Solidity developer job?
There are many ways to get a Solidity job and it might be easier than you think!
You have mastered the basics of Solidity, created your first few useful projects and now want to get your hands on some real-world projects. Getting a Solidity developer job might be easier than you think. There are generally plenty of options to choose from and often times not a lot of...
Design Pattern Solidity: Mock contracts for testing
Why you should make fun of your contracts
Mock objects are a common design pattern in object-oriented programming. Coming from the old French word 'mocquer' with the meaning of 'making fun of', it evolved to 'imitating something real' which is actually what we are doing in programming. Please only make fun of your smart contracts if you...
Kickstart your Dapp frontend development with create-eth-app
An overview on how to use the app and its features
Last time we looked at the big picture of Solidity and already mentioned the create-eth-app. Now you will find out how to use it, what features are integrated and additional ideas on how to expand on it. Started by Paul Razvan Berg, the founder of sablier, this app will kickstart your frontend...
The big picture of Solidity and Blockchain development in 2020
Overview of the most important technologies, services and tools that you need to know
Now, I do not know about you, but I remember when I first started with Solidity development being very confused by all the tools and services and how they work in connection with one another. If you are like me, this overview will help you understand the big picture of Solidity development. As I...
Design Pattern Solidity: Free up unused storage
Why you should clean up after yourself
You may or may not be used to a garbage collectors in your previous programming language. There is no such thing in Solidity and even if there was a similar concept, you would still be better off managing state data yourself. Only you as a programmer can know exactly which data will not be used...
How to setup Solidity Developer Environment on Windows
What you need to know about developing on Windows
Using Windows for development, especially for Solidity development, can be a pain sometimes, but it does not have to be. Once you have configured your environment properly, it can actually be extremely efficient and Windows is a very, very stable OS, so your overall experience can be amazing. The...
Avoiding out of gas for Truffle tests
How you do not have to worry about gas in tests anymore
You have probably seen this error message a lot of times: Error: VM Exception while processing transaction: out of gas Disclaimer : Unfortunately, this does not always actually mean what it is saying when using Truffle , especially for older versions. It can occur for various reasons and might be...
Design Pattern Solidity: Stages
How you can design stages in your contract
Closely related to the concept of finite-state machines, this pattern will help you restrict functions in your contract. You will find a lot of situations where it might be useful. Any time a contract should allow function calls only in certain stages. Let's look at an example: contract Pool {...
Web3 1.2.5: Revert reason strings
How to use the new feature
A new Web3 version was just released and it comes with a new feature that should make your life easier. With the latest version 1.2.5, you can now see the the revert reason if you use the new handleRevert option. You can activate it easily by using web3.eth.handleRevert = true . Now when you use...
Gaining back control of the internet
How Ocelot is decentralizing cloud computing
I recently came across an ambitious company that will completely redefine the way we are using the internet. Or rather, the way we are using its underlying infrastructure which ultimately is the internet. While looking at their offering, I also learned how to get anonymous cloud machines, you...
Devcon 5 - Review
Impressions from the conference
I had a lot to catch up on after Devcon. Also things didn't go quite as planned, so please excuse my delayed review! This year's Devcon was certainly stormy with a big typhoon warning already on day 1. Luckily (for us, not the people in Tokyo), it went right past Osaka. Nevertheless, a lot of...
Devcon 5 - Information, Events, Links, Telegram
What you need to know
Devcon 5 is coming up soon and there are already lots of events available, information about Osaka and more. Here is a short overview: Events Events Calendar Events Google Docs Events Kickback Most events are in all three, but if you really want to see all, you will have to look at all three...
Design Pattern Solidity: Off-chain beats on-chain
Why you should do as much as possible off-chain
As you might have realized, Ethereum transactions are anything but cheap. In particular, if you are computing complex things or storing a lot of data. That means sometimes we cannot put all logic inside Solidity. Instead, we can utilize off-chain computations to help us. A very simple example...
Design Pattern Solidity: Initialize Contract after Deployment
How to use the Initializable pattern
There are a few reasons why you might want to initialize a contract after deployment and not directly by passing constructor arguments. But first let's look at an example: contract MyCrowdsale { uint256 rate; function initialize(uint256 _rate) public { rate = _rate; } } What's the advantage over...
Consensys Blockchain Jobs Report
What the current blockchain job market looks like
Consensys published their blockchain jobs report which you can checkout in their Blockchain Developer Job Kit. The most interesting aspects are Blockchain developer jobs have been growing at a rate of 33x of the previous year according to LinkedIns jobs report Typical salary is about...
Provable — Randomness Oracle
How the Oraclize random number generator works
One particularly interesting approach by Provable is the usage of a hardware security device, namely the Ledger Nano S. It uses a trusted execution environment to generate random numbers and provides a Provable Connector Contract as interface. How to use the Provable Randomness Oracle? Use the...
Solidity Design Patterns: Multiply before Dividing
Why the correct order matters!
There has been a lot of progress since the beginning of Ethereum about best practices in Solidity. Unfortunately, I have the feeling that most of the knowledge is within the circle of experienced people and there aren’t that many online resources about it. That is why I would like to start this...
Devcon 5 Applications closing in one week
Devcon 5 Applications closing
Watch out for the Devcon 5 applications. You only have one week left to apply either as Buidler Student Scholarship Press Devcon is by far the biggest and most impressive Ethereum conference in the world. And it's full of developers! I am especially excited about the cool location this year in...
Randomness and the Blockchain
How to achieve secure randomness for Solidity smart contracts?
Update 2023 : Ethereum transitioned to Proof of Stake! If you are interested in the randomness there, you can now use the updated info over at https://soliditydeveloper.com/prevrandao. When we talk about randomness and blockchain, these are really two problems: How to generate randomness in smart...