MultiSwap: How to arbitrage with Solidity
Making multiple swaps across different decentralized exchanges in a single transaction
If you want maximum arbitrage performance, you need to swap tokens between exchanges in a single transaction. Or maybe you just want to save gas on certain swaps you perform regularly. Or maybe you have your own custom use case for swapping between decentralized exchanges. And of course maybe you are just here for the learning aspect.
Whatever your reason may be, MultiSwap is a great way to combine knowledge into one contract. Let's try to do a MultiSwap that looks like this:
So how can we achieve this?
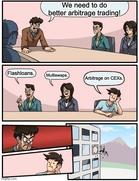
Do it manually first!
First we want to try all the trades manually. And since we are in the testing phase, we will do everything on a testnet which has deployed contracts for each protocol we want to use. In our case this happens to be only on Ropsten.
- If a similar token to what you want to trade doesn't exist on the testnet, simply deploy one yourself via Remix.
- If a token pool on a DEX doesn't exist yet on the testnet, create it yourself.
1. Banchor: ETH -> BNT
So first go to the Banchor app and swap your funds on the Ropsten network from ETH to BNT. After swapping click on the Etherscan transaction.
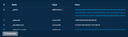
You will easily find the function name and passed parameters in the Etherscan transaction. Keep this in mind and also the documentation always helps.
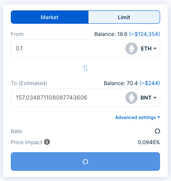
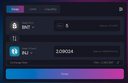
2. SushiSwap: BNT -> INJ
Then go to the SushiSwap app and swap your tokens from BNT to INJ.
Again note down the function name and parameters from the Etherscan transaction. SushiSwap is based on Uniswap 2, so you will also find more detailed explanations how it works in my previous blog post here.
3. Uniswap: INJ -> DAI
And lastly go to the Uniswap app and swap your tokens from INJ to DAI.
Again note down the function name and parameters from the Etherscan transaction. You will also find more detailed explanations how Uniswap 3 works in my previous blog post here.
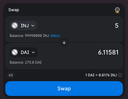
Now lets use Solidity.
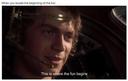
With the previous information, creating the trade logic is straight-forward. You first trade on Bancor, use the received funds to trade on SushiSwap and again use the received funds to trade on Uniswap.
1. Trading on Bancor
IBancorNetwork private constant bancorNetwork = IBancorNetwork(0xb3fa5DcF7506D146485856439eb5e401E0796B5D);
address private constant BANCOR_ETH_ADDRESS = 0xEeeeeEeeeEeEeeEeEeEeeEEEeeeeEeeeeeeeEEeE;
address private constant BANCOR_ETHBNT_POOL = 0x1aCE5DD13Ba14CA42695A905526f2ec366720b13;
address private constant BNT = 0xF35cCfbcE1228014F66809EDaFCDB836BFE388f5;
function _tradeOnBancor(uint256 amountIn, uint256 amountOutMin) private {
bancorNetwork.convertByPath{value: msg.value}(_getPathForBancor(), amountIn, amountOutMin, address(0), address(0), 0);
}
function _getPathForBancor() private pure returns (address[] memory) {
address[] memory path = new address[](3);
path[0] = BANCOR_ETH_ADDRESS;
path[1] = BANCOR_ETHBNT_POOL;
path[2] = BNT;
return path;
}
Our function to trade on Banchor is basically self-explanatory. We obtained the addresses for the path and bancor network from our example transaction.
2. Trading on Sushi
IUniswapV2Router02 private constant sushiRouter = IUniswapV2Router02(0x1b02dA8Cb0d097eB8D57A175b88c7D8b47997506);
address private constant INJ = 0x9108Ab1bb7D054a3C1Cd62329668536f925397e5;
function _tradeOnSushi(uint256 amountIn, uint256 amountOutMin, uint256 deadline) private {
address recipient = address(this);
sushiRouter.swapExactTokensForTokens(
amountIn,
amountOutMin,
_getPathForSushiSwap(),
recipient,
deadline
);
}
function _getPathForSushiSwap() private pure returns (address[] memory) {
address[] memory path = new address[](2);
path[0] = BNT;
path[1] = INJ;
return path;
}
Then we can use swapExactTokensForTokens to swap BNT to INJ. The path simply consists of the tokens. We received the router address from our example transaction.
3. Trading on Uniswap
IUniswapRouter private constant uniswapRouter = IUniswapRouter(0xE592427A0AEce92De3Edee1F18E0157C05861564);
address private constant DAI = 0xaD6D458402F60fD3Bd25163575031ACDce07538D;
function _tradeOnUniswap(uint256 amountIn, uint256 amountOutMin, uint256 deadline) private {
address tokenIn = INJ;
address tokenOut = DAI;
uint24 fee = 3000;
address recipient = msg.sender;
uint160 sqrtPriceLimitX96 = 0;
ISwapRouter.ExactInputSingleParams memory params = ISwapRouter.ExactInputSingleParams(
tokenIn,
tokenOut,
fee,
recipient,
deadline,
amountIn,
amountOutMin,
sqrtPriceLimitX96
);
uniswapRouter.exactInputSingle(params);
uniswapRouter.refundETH();
// refund leftover ETH to user
(bool success,) = msg.sender.call{ value: address(this).balance }("");
require(success, "refund failed");
}
4. Bringing it all together
We need to approve the SushiSwap contract to use our BNT and the Uniswap contract to use our INJ. It's more gas-efficient to do this only once on the deployment, so put it in your constructor:
constructor() {
IERC20(BNT).safeApprove(address(sushiRouter), type(uint256).max);
IERC20(INJ).safeApprove(address(uniswapRouter), type(uint256).max);
}
Now we have everything we need. Let's create a multiSwap
function.
function multiSwap(uint256 deadline, uint256 amountOutMinUniswap) external payable {
uint256 amountOutMinBancor = 1;
uint256 amountOutMinSushiSwap = 1;
_tradeOnBancor(msg.value, amountOutMinBancor);
_tradeOnSushi(IERC20(BNT).balanceOf(address(this)), amountOutMinSushiSwap, deadline);
_tradeOnUniswap(IERC20(INJ).balanceOf(address(this)), amountOutMinUniswap, deadline);
}
As you can see swapping the tokens now is easy. For Bancor and SushiSwap we don't care how many tokens we receive, so we put the min values to 1. The only thing that matters is how many DAI tokens we receive in the last swap. This value is passed from the outside as well as the deadline as a UNIX timestamp. If you don't care when the trade is executed, simply pass a high deadline value.
Now how do you obtain a reasonable amountOutMinUniswap
value? To help us here we can create a second function only meant to be called as view function:
// meant to be called as view function
function multiSwapPreview() external payable returns(uint256) {
uint256 daiBalanceUserBeforeTrade = IERC20(DAI).balanceOf(msg.sender);
uint256 deadline = block.timestamp + 300;
uint256 amountOutMinBancor = 1;
uint256 amountOutMinSushiSwap = 1;
uint256 amountOutMinUniswap = 1;
_tradeOnBancor(msg.value, amountOutMinBancor);
_tradeOnSushi(IERC20(BNT).balanceOf(address(this)), amountOutMinSushiSwap, deadline);
_tradeOnUniswap(IERC20(INJ).balanceOf(address(this)), amountOutMinUniswap, deadline);
uint256 daiBalanceUserAfterTrade = IERC20(DAI).balanceOf(msg.sender);
return daiBalanceUserAfterTrade - daiBalanceUserBeforeTrade;
}
But note that we didn't declare this as a view function, but do not call this function on-chain. It's still meant to be called as a view function, but since it's using non-view functions under the hood to compute the result, it's not possible to declare it a view function itself (Solidity feature request?). Use for example Web3's call() functionality to read the result in the frontend.
Now we can call multiSwapPreview
in our frontend. To increase the chances that the transaction won't get reverted, we can decrease the estimated amount of DAI received by a little bit:
const estimatedDAI = (await myContract.multiSwapPreview({ value: ethAmount }).call())[0];
const amountOutMinUniswap = estimatedDAI * 0.96;
Now we only need one transaction for the whole swap!
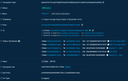
You can find a fully working example for the trade here. Once you have mastered it on a testnet, you can repeat the process for mainnet. If you dont want to spend extra ETH for the manual transactions, you can inspect the transaction data and contract address before submitting anything. You mostly just need to change the contract address.
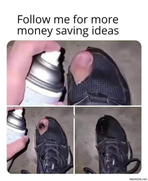
Solidity Developer
More great blog posts from Markus Waas
How to use ChatGPT with Solidity
Using the Solidity Scholar and other GPT tips
How to integrate Uniswap 4 and create custom hooks
Let's dive into Uniswap v4's new features and integration
How to integrate Wormhole in your smart contracts
Entering a New Era of Blockchain Interoperability
Solidity Deep Dive: New Opcode 'Prevrandao'
All you need to know about the latest opcode addition
How Ethereum scales with Arbitrum Nitro and how to use it
A blockchain on a blockchain deep dive
The Ultimate Merkle Tree Guide in Solidity
Everything you need to know about Merkle trees and their future
The New Decentralized The Graph Network
What are the new features and how to use it
zkSync Guide - The future of Ethereum scaling
How the zero-knowledge tech works and how to use it
Exploring the Openzeppelin CrossChain Functionality
What is the new CrossChain support and how can you use it.
Deploying Solidity Contracts in Hedera
What is Hedera and how can you use it.
Writing ERC-20 Tests in Solidity with Foundry
Blazing fast tests, no more BigNumber.js, only Solidity
ERC-4626: Extending ERC-20 for Interest Management
How the newly finalized standard works and can help you with Defi
Advancing the NFT standard: ERC721-Permit
And how to avoid the two step approve + transferFrom with ERC721-Permit (EIP-4494)
Moonbeam: The EVM of Polkadot
Deploying and onboarding users to Moonbeam or Moonriver
Advanced MultiSwap: How to better arbitrage with Solidity
Making multiple swaps across different decentralized exchanges in a single transaction
Deploying Solidity Smart Contracts to Solana
What is Solana and how can you deploy Solidity smart contracts to it?
Smock 2: The powerful mocking tool for Hardhat
Features of smock v2 and how to use them with examples
How to deploy on Evmos: The first EVM chain on Cosmos
Deploying and onboarding users to Evmos
EIP-2535: A standard for organizing and upgrading a modular smart contract system.
Multi-Facet Proxies for full control over your upgrades
The latest tech for scaling your contracts: Optimism
How the blockchain on a blockchain works and how to use it
Ultimate Performance: The Aurora Layer2 Network
Deploying and onboarding users to the Aurora Network powered by NEAR Protocol
What is ecrecover in Solidity?
A dive into the waters of signatures for smart contracts
How to use Binance Smart Chain in your Dapp
Deploying and onboarding users to the Binance Smart Chain (BSC)
Using the new Uniswap v3 in your contracts
What's new in Uniswap v3 and how to integrate Uniswap v3
What's coming in the London Hardfork?
Looking at all the details of the upcoming fork
Welcome to the Matrix of blockchain
How to get alerted *before* getting hacked and prevent it
The Ultimate Ethereum Mainnet Deployment Guide
All you need to know to deploy to the Ethereum mainnet
SushiSwap Explained!
Looking at the implementation details of SushiSwap
Solidity Fast Track 2: Continue Learning Solidity Fast
Continuing to learn Solidity fast with the advanced basics
What's coming in the Berlin Hardfork?
Looking at all the details of the upcoming fork
Using 1inch ChiGas tokens to reduce transaction costs
What are gas tokens and example usage for Uniswap v2
Openzeppelin Contracts v4 in Review
Taking a look at the new Openzeppelin v4 Release
EIP-3156: Creating a standard for Flash Loans
A new standard for flash loans unifying the interface + wrappers for existing ecosystems
Tornado.cash: A story of anonymity and zk-SNARKs
What is Tornado.cash, how to use it and the future
High Stakes Roulette on Ethereum
Learn by Example: Building a secure High Stakes Roulette
How to implement generalized meta transactions
We'll explore a powerful design for meta transactions based on 0x
Utilizing Bitmaps to dramatically save on Gas
A simple pattern which can save you a lot of money
Using the new Uniswap v2 as oracle in your contracts
How does the Uniswap v2 oracle function and how to integrate with it
Smock: The powerful mocking tool for Hardhat
Features of smock and how to use them with examples
How to build and use ERC-721 tokens in 2021
An intro for devs to the uniquely identifying token standard and its future
Trustless token management with Set Protocol
How to integrate token sets in your contracts
Exploring the new Solidity 0.8 Release
And how to upgrade your contracts to Solidity 0.8
How to build and use ERC-1155 tokens
An intro to the new standard for having many tokens in one
Leveraging the power of Bitcoins with RSK
Learn how RSK works and how to deploy your smart contracts to it
Solidity Fast Track: Learn Solidity Fast
'Learn X in Y minutes' this time with X = Solidity 0.7 and Y = 20
Sourcify: The future of a Decentralized Etherscan
Learn how to use the new Sourcify infrastructure today
Integrating the 0x API into your contracts
How to automatically get the best prices via 0x
How to build and use ERC-777 tokens
An intro to the new upgraded standard for ERC-20 tokens
COMP Governance Explained
How Compound's Decentralized Governance is working under the hood
How to prevent stuck tokens in contracts
And other use cases for the popular EIP-165
Understanding the World of Automated Smart Contract Analyzers
What are the best tools today and how can you use them?
A Long Way To Go: On Gasless Tokens and ERC20-Permit
And how to avoid the two step approve + transferFrom with ERC20-Permit (EIP-2612)!
Smart Contract Testing with Waffle 3
What are the features of Waffle and how to use them.
How to use xDai in your Dapp
Deploying and onboarding users to xDai to avoid the high gas costs
Stack Too Deep
Three words of horror
Integrating the new Chainlink contracts
How to use the new price feeder oracles
TheGraph: Fixing the Web3 data querying
Why we need TheGraph and how to use it
Adding Typescript to Truffle and Buidler
How to use TypeChain to utilize the powers of Typescript in your project
Integrating Balancer in your contracts
What is Balancer and how to use it
Navigating the pitfalls of securely interacting with ERC20 tokens
Figuring out how to securely interact might be harder than you think
Why you should automatically generate interests from user funds
How to integrate Aave and similar systems in your contracts
How to use Polygon (Matic) in your Dapp
Deploying and onboarding users to Polygon to avoid the high gas costs
Migrating from Truffle to Buidler
And why you should probably keep both.
Contract factories and clones
How to deploy contracts within contracts as easily and gas-efficient as possible
How to use IPFS in your Dapp?
Using the interplanetary file system in your frontend and contracts
Downsizing contracts to fight the contract size limit
What can you do to prevent your contracts from getting too large?
Using EXTCODEHASH to secure your systems
How to safely integrate anyone's smart contract
Using the new Uniswap v2 in your contracts
What's new in Uniswap v2 and how to integrate Uniswap v2
Solidity and Truffle Continuous Integration Setup
How to setup Travis or Circle CI for Truffle testing along with useful plugins.
Upcoming Devcon 2021 and other events
The Ethereum Foundation just announced the next Devcon in 2021 in Colombia
The Year of the 20: Creating an ERC20 in 2020
How to use the latest and best tools to create an ERC-20 token contract
How to get a Solidity developer job?
There are many ways to get a Solidity job and it might be easier than you think!
Design Pattern Solidity: Mock contracts for testing
Why you should make fun of your contracts
Kickstart your Dapp frontend development with create-eth-app
An overview on how to use the app and its features
The big picture of Solidity and Blockchain development in 2020
Overview of the most important technologies, services and tools that you need to know
Design Pattern Solidity: Free up unused storage
Why you should clean up after yourself
How to setup Solidity Developer Environment on Windows
What you need to know about developing on Windows
Avoiding out of gas for Truffle tests
How you do not have to worry about gas in tests anymore
Design Pattern Solidity: Stages
How you can design stages in your contract
Web3 1.2.5: Revert reason strings
How to use the new feature
Gaining back control of the internet
How Ocelot is decentralizing cloud computing
Devcon 5 - Review
Impressions from the conference
Devcon 5 - Information, Events, Links, Telegram
What you need to know
Design Pattern Solidity: Off-chain beats on-chain
Why you should do as much as possible off-chain
Design Pattern Solidity: Initialize Contract after Deployment
How to use the Initializable pattern
Consensys Blockchain Jobs Report
What the current blockchain job market looks like
Provable — Randomness Oracle
How the Oraclize random number generator works
Solidity Design Patterns: Multiply before Dividing
Why the correct order matters!
Devcon 5 Applications closing in one week
Devcon 5 Applications closing
Randomness and the Blockchain
How to achieve secure randomness for Solidity smart contracts?