A Long Way To Go: On Gasless Tokens and ERC20-Permit
And how to avoid the two step approve + transferFrom with ERC20-Permit (EIP-2612)!
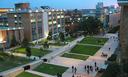
It's April 2019 in Sydney. Here I am looking for the Edcon Hackathon inside the massive Sydney university complex. It feels like a little city within a city. Of course, I am at the wrong end of the complex and I realize to get to the venue hosting the Hackathon I need to walk 30 minutes to the other side. At the venue I register just a few minutes before the official start!
With all participants living and breathing crypto, a system was setup which allowed payments with DAI in one of the cafeterias. This is particularly useful, because there is also a promotion running by AlphaWallet giving away 20 promotional DAI to Hackathon participants (and later on discounted drinks). With my wallet already downloaded and 20 DAI, it's the perfect time to find the cafeteria...
Not so easy as it turns out. Firstly, it's a 15 minute walk back to the center of the university city. I finally find it. I choose my lunch and I'm happy to try this new payment system. I've paid with Bitcoin in restaurants before back in 2012, but this would be my first time using ERC-20. I scan the QR-code, enter the amount in DAI to pay and...
'Not enough gas available to cover the transaction fees.'
Yikes! All the excitement gone. Of course you need ETH to pay for the gas! And my new wallet had 0 ETH. I'm a Solidity developer, I know this. Yet it happened even to me. My computer with ETH on it was all the way back at the venue, so there was no solution for me. Without lunch in my hands taking the long walk back to the venue, I thought to myself; we have a long way to go for this technology to become more mainstream.
Fast forward to EIP-2612
Since then, DAI and Uniswap have lead the way towards a new standard named EIP-2612 which can get rid of the approve + transferFrom, while also allowing gasless token transfers. DAI was the first to add a new permit
function to its ERC-20 token. It allows a user to sign an approve transaction off-chain producing a signature that anyone could use and submit to the blockchain. It's a fundamental first step towards solving the gas payment issue and also removes the user-unfriendly 2-step process of sending approve
and later transferFrom
.
Let's examine the EIP in detail.
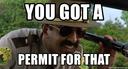
Naive Incorrect Approach
On a high level the procedure is very simple. Instead of a user signing an approve transaction, he signs the data "approve(spender, amount)". The result can be passed by anyone to the permit
function where we simply retrieve the signer address using ecrecover
, followed by approve(signer, spender, amount)
.
This construction can be used to allow someone else to pay for the gas costs and also to remove the common approve + transferFrom pattern:
Before:
- User submits
token.approve(myContract.address, amount)
transaction. - Wait for transaction confirmation.
- User submits second
myContract.doSomething()
transaction which internally usestoken.transferFrom
.
After:
- User signs
signature = approve(myContract.address, amount)
. - User submits signature to
myContract.doSomething(signature)
. myContract
usestoken.permit
to increase allowance, followed bytoken.transferFrom
.
We go from two transaction submissions, to only one!
Permit in Detail: Preventing Misuse and Replays
The main issue we are facing is that a valid signature might be used several times or in other places where it's not intended to be used in. To prevent this we are adding several parameters. Under the hood we are using the already existing, widely used EIP-712 standard.
1. EIP-712 Domain Hash
With EIP-712, we define a domain separator for our ERC-20:
bytes32 eip712DomainHash = keccak256(
abi.encode(
keccak256(
"EIP712Domain(string name,string version,uint256 chainId,address verifyingContract)"
),
keccak256(bytes(name())), // ERC-20 Name
keccak256(bytes("1")), // Version
chainid(),
address(this)
)
);
This ensures a signature is only used for our given token contract address on the correct chain id. The chain id was introduced to exactly identify a network after the Ethereum Classic fork which continued to use a network id of 1. A list of existing chain ids can be seen here.
2. Permit Hash Struct
Now we can create a Permit specific signature:
bytes32 hashStruct = keccak256(
abi.encode(
keccak256("Permit(address owner,address spender,uint256 value,uint256 nonce,uint256 deadline)"),
owner,
spender,
amount,
nonces[owner],
deadline
)
);
This hashStruct will ensure that the signature can only used for
- the
permit
function - to approve from
owner
- to approve for
spender
- to approve the given
value
- only valid before the given
deadline
- only valid for the given
nonce
The nonce ensures someone can not replay a signature, i.e., use it multiple times on the same contract.
3. Final Hash
bytes32 hash = keccak256(
abi.encodePacked(uint16(0x1901), eip712DomainHash, hashStruct)
);
4. Verifying the Signature
Using this hash we can use ecrecover to retrieve the signer of the function:
address signer = ecrecover(hash, v, r, s);
require(signer == owner, "ERC20Permit: invalid signature");
require(signer != address(0), "ECDSA: invalid signature");
Invalid signatures will produce an empty address, that's what the last check is for.
5. Increasing Nonce and Approving
Now lastly we only have to increase the nonce for the owner and call the approve function:
nonces[owner]++;
_approve(owner, spender, amount);
You can see a full implementation example here.
Existing ERC20-Permit Implementations
DAI ERC20-Permit
permit
as described here. The implementation differs from EIP-2612 slightly- instead of
value
, it only takes a boolallowed
and sets the allowance either to0
orMAX_UINT256
- the
deadline
parameter is calledexpiry
Uniswap ERC20-Permit
The Uniswap implementation aligns with the current EIP-2612, see here. It allows you to call removeLiquidityWithPermit, removing the additional approve
step.
If you want to get a feel for the process, go to https://app.uniswap.org/#/pool and change to the Kovan network. Not add liquidity to a pool. Now try to remove it. After clicking on 'Approve', you will notice this MetaMask popup as show on the right.
This will not submit a transaction, but only creates a signature with the given parameters. You can sign it and in a second step call removeLiquidityWithPermit with the previously generated signature. All in all: just one transaction submission.
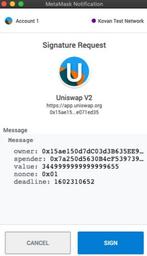
ERC20-Permit Library
I have created an ERC-20 Permit library that you can import. You can find it at https://github.com/soliditylabs/ERC20-Permit.
Built using
- OpenZeppelin ERC20-Permit
- 0x-inspired gas saving hashes with assembly
- eth-permit frontend library for testing
You can simply use it by installing via npm:
$ npm install @soliditylabs/erc20-permit --save-dev
Import it into your ERC-20 contract like this:
// SPDX-License-Identifier: MIT
pragma solidity ^0.7.0;
import {ERC20, ERC20Permit} from "@soliditylabs/erc20-permit/contracts/ERC20Permit.sol";
contract ERC20PermitToken is ERC20Permit {
constructor (uint256 initialSupply) ERC20("ERC20Permit-Token", "EPT") {
_mint(msg.sender, initialSupply);
}
}
Frontend Usage
You can see here in my tests how I use the eth-permit
library to create valid signatures. It automatically fetches the correct nonce and sets the parameters according to the current standard. It also supports the DAI-style permit signature creation. Full documentation available at https://github.com/dmihal/eth-permit.
A word on debugging: It can be painful. Any single parameter off will result in a revert: Invalid signature
. Good luck finding out the reason why.
At the time of this writing, there still seems to be an open issue with it which may or may not affect you depending on your Web3 provider. If it does affect you, just use my patch here installed via patch-package.
Solution for Gasless Tokens
Now recall my Sydney experience. This standard alone wouldn't solve the problem, but it's first basic module towards it. Now you can create a Gas Station Network such as Open GSN. Deploying contracts for it that simply transfer the tokens via permit + transferFrom. And nodes running inside the GSN will take the permit signatures and submit them.
Who pays the gas fees? That will depend on the specific use case. Maybe the Dapp company pays the fees as part of their customer acquisition cost (CAC). Maybe the GSN nodes are paid by the transferred tokens. We still have a long way to go to figure out all the details.
As always use with care
Be aware that the standard is not yet final. It's currently identical to the Uniswap implementation, but it may or may not change in the future. I will keep the library updated in case the standard changes again. My library code was also not audited, use at your own risk.
You have reached the end of the article. I hereby permit you to comment and ask questions.
Solidity Developer
More great blog posts from Markus Waas
How to use ChatGPT with Solidity
Using the Solidity Scholar and other GPT tips
How to integrate Uniswap 4 and create custom hooks
Let's dive into Uniswap v4's new features and integration
How to integrate Wormhole in your smart contracts
Entering a New Era of Blockchain Interoperability
Solidity Deep Dive: New Opcode 'Prevrandao'
All you need to know about the latest opcode addition
How Ethereum scales with Arbitrum Nitro and how to use it
A blockchain on a blockchain deep dive
The Ultimate Merkle Tree Guide in Solidity
Everything you need to know about Merkle trees and their future
The New Decentralized The Graph Network
What are the new features and how to use it
zkSync Guide - The future of Ethereum scaling
How the zero-knowledge tech works and how to use it
Exploring the Openzeppelin CrossChain Functionality
What is the new CrossChain support and how can you use it.
Deploying Solidity Contracts in Hedera
What is Hedera and how can you use it.
Writing ERC-20 Tests in Solidity with Foundry
Blazing fast tests, no more BigNumber.js, only Solidity
ERC-4626: Extending ERC-20 for Interest Management
How the newly finalized standard works and can help you with Defi
Advancing the NFT standard: ERC721-Permit
And how to avoid the two step approve + transferFrom with ERC721-Permit (EIP-4494)
Moonbeam: The EVM of Polkadot
Deploying and onboarding users to Moonbeam or Moonriver
Advanced MultiSwap: How to better arbitrage with Solidity
Making multiple swaps across different decentralized exchanges in a single transaction
Deploying Solidity Smart Contracts to Solana
What is Solana and how can you deploy Solidity smart contracts to it?
Smock 2: The powerful mocking tool for Hardhat
Features of smock v2 and how to use them with examples
How to deploy on Evmos: The first EVM chain on Cosmos
Deploying and onboarding users to Evmos
EIP-2535: A standard for organizing and upgrading a modular smart contract system.
Multi-Facet Proxies for full control over your upgrades
MultiSwap: How to arbitrage with Solidity
Making multiple swaps across different decentralized exchanges in a single transaction
The latest tech for scaling your contracts: Optimism
How the blockchain on a blockchain works and how to use it
Ultimate Performance: The Aurora Layer2 Network
Deploying and onboarding users to the Aurora Network powered by NEAR Protocol
What is ecrecover in Solidity?
A dive into the waters of signatures for smart contracts
How to use Binance Smart Chain in your Dapp
Deploying and onboarding users to the Binance Smart Chain (BSC)
Using the new Uniswap v3 in your contracts
What's new in Uniswap v3 and how to integrate Uniswap v3
What's coming in the London Hardfork?
Looking at all the details of the upcoming fork
Welcome to the Matrix of blockchain
How to get alerted *before* getting hacked and prevent it
The Ultimate Ethereum Mainnet Deployment Guide
All you need to know to deploy to the Ethereum mainnet
SushiSwap Explained!
Looking at the implementation details of SushiSwap
Solidity Fast Track 2: Continue Learning Solidity Fast
Continuing to learn Solidity fast with the advanced basics
What's coming in the Berlin Hardfork?
Looking at all the details of the upcoming fork
Using 1inch ChiGas tokens to reduce transaction costs
What are gas tokens and example usage for Uniswap v2
Openzeppelin Contracts v4 in Review
Taking a look at the new Openzeppelin v4 Release
EIP-3156: Creating a standard for Flash Loans
A new standard for flash loans unifying the interface + wrappers for existing ecosystems
Tornado.cash: A story of anonymity and zk-SNARKs
What is Tornado.cash, how to use it and the future
High Stakes Roulette on Ethereum
Learn by Example: Building a secure High Stakes Roulette
How to implement generalized meta transactions
We'll explore a powerful design for meta transactions based on 0x
Utilizing Bitmaps to dramatically save on Gas
A simple pattern which can save you a lot of money
Using the new Uniswap v2 as oracle in your contracts
How does the Uniswap v2 oracle function and how to integrate with it
Smock: The powerful mocking tool for Hardhat
Features of smock and how to use them with examples
How to build and use ERC-721 tokens in 2021
An intro for devs to the uniquely identifying token standard and its future
Trustless token management with Set Protocol
How to integrate token sets in your contracts
Exploring the new Solidity 0.8 Release
And how to upgrade your contracts to Solidity 0.8
How to build and use ERC-1155 tokens
An intro to the new standard for having many tokens in one
Leveraging the power of Bitcoins with RSK
Learn how RSK works and how to deploy your smart contracts to it
Solidity Fast Track: Learn Solidity Fast
'Learn X in Y minutes' this time with X = Solidity 0.7 and Y = 20
Sourcify: The future of a Decentralized Etherscan
Learn how to use the new Sourcify infrastructure today
Integrating the 0x API into your contracts
How to automatically get the best prices via 0x
How to build and use ERC-777 tokens
An intro to the new upgraded standard for ERC-20 tokens
COMP Governance Explained
How Compound's Decentralized Governance is working under the hood
How to prevent stuck tokens in contracts
And other use cases for the popular EIP-165
Understanding the World of Automated Smart Contract Analyzers
What are the best tools today and how can you use them?
Smart Contract Testing with Waffle 3
What are the features of Waffle and how to use them.
How to use xDai in your Dapp
Deploying and onboarding users to xDai to avoid the high gas costs
Stack Too Deep
Three words of horror
Integrating the new Chainlink contracts
How to use the new price feeder oracles
TheGraph: Fixing the Web3 data querying
Why we need TheGraph and how to use it
Adding Typescript to Truffle and Buidler
How to use TypeChain to utilize the powers of Typescript in your project
Integrating Balancer in your contracts
What is Balancer and how to use it
Navigating the pitfalls of securely interacting with ERC20 tokens
Figuring out how to securely interact might be harder than you think
Why you should automatically generate interests from user funds
How to integrate Aave and similar systems in your contracts
How to use Polygon (Matic) in your Dapp
Deploying and onboarding users to Polygon to avoid the high gas costs
Migrating from Truffle to Buidler
And why you should probably keep both.
Contract factories and clones
How to deploy contracts within contracts as easily and gas-efficient as possible
How to use IPFS in your Dapp?
Using the interplanetary file system in your frontend and contracts
Downsizing contracts to fight the contract size limit
What can you do to prevent your contracts from getting too large?
Using EXTCODEHASH to secure your systems
How to safely integrate anyone's smart contract
Using the new Uniswap v2 in your contracts
What's new in Uniswap v2 and how to integrate Uniswap v2
Solidity and Truffle Continuous Integration Setup
How to setup Travis or Circle CI for Truffle testing along with useful plugins.
Upcoming Devcon 2021 and other events
The Ethereum Foundation just announced the next Devcon in 2021 in Colombia
The Year of the 20: Creating an ERC20 in 2020
How to use the latest and best tools to create an ERC-20 token contract
How to get a Solidity developer job?
There are many ways to get a Solidity job and it might be easier than you think!
Design Pattern Solidity: Mock contracts for testing
Why you should make fun of your contracts
Kickstart your Dapp frontend development with create-eth-app
An overview on how to use the app and its features
The big picture of Solidity and Blockchain development in 2020
Overview of the most important technologies, services and tools that you need to know
Design Pattern Solidity: Free up unused storage
Why you should clean up after yourself
How to setup Solidity Developer Environment on Windows
What you need to know about developing on Windows
Avoiding out of gas for Truffle tests
How you do not have to worry about gas in tests anymore
Design Pattern Solidity: Stages
How you can design stages in your contract
Web3 1.2.5: Revert reason strings
How to use the new feature
Gaining back control of the internet
How Ocelot is decentralizing cloud computing
Devcon 5 - Review
Impressions from the conference
Devcon 5 - Information, Events, Links, Telegram
What you need to know
Design Pattern Solidity: Off-chain beats on-chain
Why you should do as much as possible off-chain
Design Pattern Solidity: Initialize Contract after Deployment
How to use the Initializable pattern
Consensys Blockchain Jobs Report
What the current blockchain job market looks like
Provable — Randomness Oracle
How the Oraclize random number generator works
Solidity Design Patterns: Multiply before Dividing
Why the correct order matters!
Devcon 5 Applications closing in one week
Devcon 5 Applications closing
Randomness and the Blockchain
How to achieve secure randomness for Solidity smart contracts?